Overview
Internship Diary is a desktop internship tracking application written in Java, and has about 15 kLoC. The user interacts with it using a CLI, and it has a GUI created with JavaFX.
Summary of contributions
-
Major enhancement: Revamp the find command
-
What it does: This enhancement enables the general find where the keywords that the user entered will list any internship application that has fields that matches any of the keywords provided. This enhancement also enables finding by specific field(s), where the user can specify which field(s) they are interested in searching in. Only the internship applications that has the field(s) matching the specified field(s) keywords will be listed in this case.
-
Justification: This feature will allow the user to easily filter the list to quickly get to what is important. With the flexibility of general and specific find, the user can get a more specific filtered list if needed.
-
Highlights: Quite a bit of planning was involved, mainly to consider how the search should behave and how it is performed. It the end, the plan to use predicates was decided as it will be easy to extend the application with minimal changes in the command. Quite a bit of knowledge on how predicates work is also required as there was a need to find out how to combine many predicates cleanly so that it is readable and to ensure that it is working correctly. There was also a need decide on how I should allow the user to tell the system which of the two types of find command to invoke. It was decided in the end to use the same command word and have the type of user input be the way of telling the system which type of find to invoke.
-
-
Minor enhancement: Add visual display of upcoming application deadlines and interviews and inactive applications
-
What it does: This enhancement allows the user to determine which application has deadlines or interviews due in 7 days or if there are ghosted application by the color of the internship application in the list. Internship applications with upcoming deadline or interview will show green while ghosted applications will show red.
-
Justification: This enhancement will allow the user to know what is upcoming and what is ghosted/inactive at a quick glance.
-
Highlights: Figuring out where the code to update the color should go was a challenge. Setting it directly in CSS was not possible as I still wanted to keep the odd and even colors for the list. When setting it in code, I had to figure out why the style will not revert to the default CSS style, leading to visual bugs. The bugs were fixed after some research.
-
-
Code contributed: RepoSense
-
Other contributions:
-
Project management:
-
Managed releases
v1.2
-v1.4
(3 releases) on GitHub
-
-
Enhancement to existing features:
-
Documentation:
-
Helped with the refactoring of the developer guide from AB3 to Internship Diary: (PRs: #20)
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Locating internship applications: find
Finds all internship application(s) with the specified fields containing any of the given keywords.
The find parameters will appear at the bottom left of the internship diary |
Format: find [KEYWORDS] [c/COMPANY] [r/ROLE] [a/ADDRESS] [p/PHONE] [e/EMAIL] [d/DATE] [w/PRIORITY] [s/STATUS]
Example(s):
-
find Google
Lists internship application(s) with companyGoogle
OR with emailalice@google.com
.
![]() Figure 1. Before executing
find Google . |
![]() Figure 2. After executing
find Google . |
-
find c/Google s/applied
Lists internship application(s) with companyGoogle
AND statusapplied
.
![]() Figure 3. Before executing
find c/Google s/applied . |
![]() Figure 4. After executing
find c/Google s/applied . |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Find Command
The find command allows the user to get a filtered list of internship applications. The following sequence diagram will illustrate the process of invocation for the command:
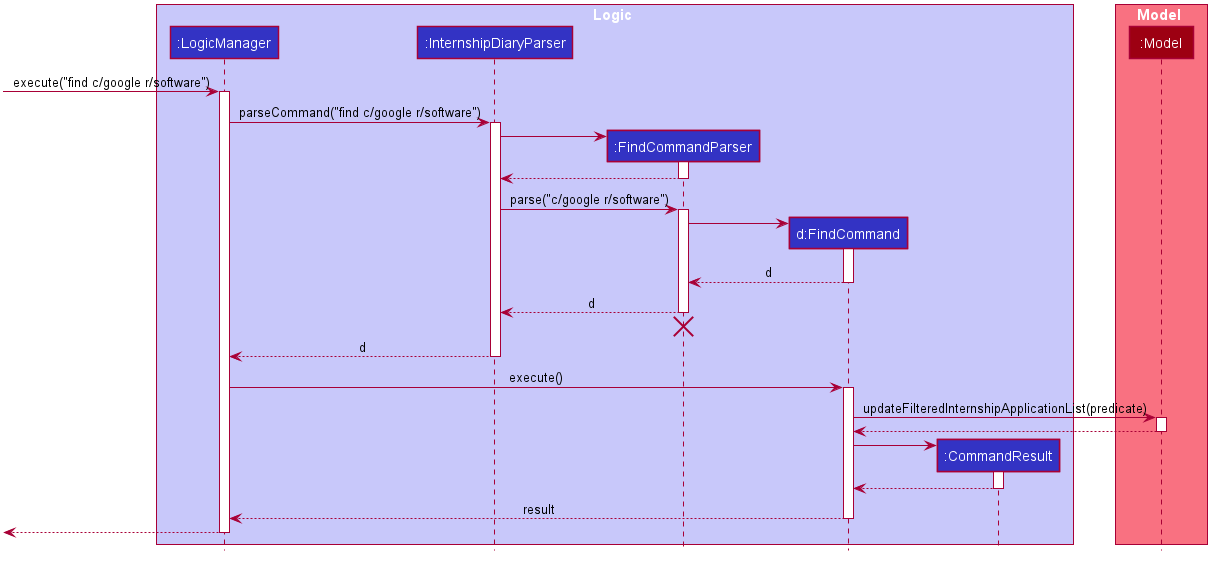
find c/google r/software
commandThe following subsections will go through the general implementations of the find command, as well as the 2 versions of the command, find any match, and find match by fields.
Implementation
The find command is implemented in the class FindCommand
and uses the FindCommandParser
class to parse the arguments for the command.
To facilitate the find command, several predicates classes implementing Predicate<InternshipApplication>
are used:
-
CompanyContainsKeywordsPredicate
— Predicate to check if an internship application’sCompany
field contains any substring matching any words in the list supplied by its constructorCompanyContainsKeywordsPredicate(List<String> keywords)
. -
RoleContainsKeywordsPredicate
— Predicate to check if an internship application’sRole
field contains any substring matching any words in the list supplied by its constructorRoleContainsKeywordsPredicate(List<String> keywords)
. -
AddressContainsKeywordsPredicate
— Predicate to check if an internship application’sAddress
field contains any substring matching any words in the list supplied by its constructorAddressContainsKeywordsPredicate(List<String> keywords)
. -
PhoneContainsNumbersPredicate
— Predicate to check if an internship application’sPhone
field contains any substring matching any words in the list supplied by its constructorPhoneContainsNumbersPredicate(List<String> numbers)
. -
EmailContainsKeywordsPredicate
— Predicate to check if an internship application’sEmail
field contains any substring matching any words in the list supplied by its constructorEmailContainsKeywordsPredicate(List<String> keywords)
. -
PriorityContainsNumbersPredicate
— Predicate to check if an internship application’sPriority
field exactly matches any words in the list supplied by its constructorPriorityContainsNumbersPredicate(List<String> numbers)
. -
ApplicationDateIsDatePredicate
— Predicate to check if an internship application’sApplicationDate
field is exactly the date supplied by its constructorApplicationDateIsDatePredicate(List<String> dateArr)
. The constructor will parsedateArr
into aLocalDate
. -
StatusContainsKeywordsPredicate
— Predicate to check if an internship application’sStatus
field contains any substring matching any words in the list supplied by its constructorStatusContainsKeywordsPredicate(List<String> keywords)
.
The following class diagram show the relationship of the above mentioned predicates, Predicate
, FindCommandParser
and FindCommand
:
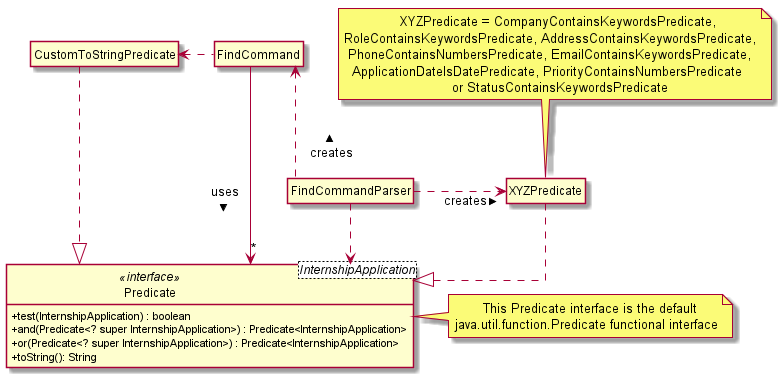
Predicates
, FindCommandParser
and FindCommand
Find Any Match
This version of the command is invoked when the user enters the command with preamble text in the parameter, e.g.
find google facebook
or find google r/software
.
The command will perform search for any internship application where any of the fields Company
, Role
, Address
, Phone
, Email
, Priority
or Status
contains a substring matching at least one word in the preamble and display them, e.g. find google facebook
will look for internship applications whose any of the above fields contains the substring google
or facebook
.
The searching and displaying of the internship application is done by performing an OR
operation on all the predicates
CompanyContainsKeywordsPredicate
, RoleContainsKeywordsPredicate
, AddressContainsKeywordsPredicate
,
PhoneContainsNumbersPredicate
, EmailContainsKeywordsPredicate
, PriorityContainsNumbersPredicate
and
StatusContainsKeywordsPredicate
to get a single predicate and passing that into the method
updateFilteredInternshipApplicationList()
of the ModelManager
instance.
Find Match by Fields
This version of the command is invoked when the user enters the command without any preamble text in the parameter, e.g.
find c/google r/software
.
The command will perform a search for any internship application where the fields
Company
, Role
, Address
, Phone
, Email
, ApplicationDate
, Priority
and Status
match any of the supplied word after their respective prefixes (if a field’s prefix is not specified, the field is not checked), e.g. find c/google facebook d/01 02 2020
will look for internship applications where the Company
field contains a substring google
or facebook
and the ApplicationDate
field matching the date 1st February 2020.
The searching and displaying of the internship application is done by performing an AND
operation on the required predicates that is any of CompanyContainsKeywordsPredicate
, RoleContainsKeywordsPredicate
,
AddressContainsKeywordsPredicate
, PhoneContainsNumbersPredicate
, EmailContainsKeywordsPredicate
,
ApplicationDateIsDatePredicate
, PriorityContainsNumbersPredicate
and StatusContainsKeywordsPredicate
to get a single predicate and passing that into the method updateFilteredInternshipApplicationList()
of the ModelManager
instance.
Determine which Find to Invoke
The following activity diagram summarises how which type of find to invoke is determined:
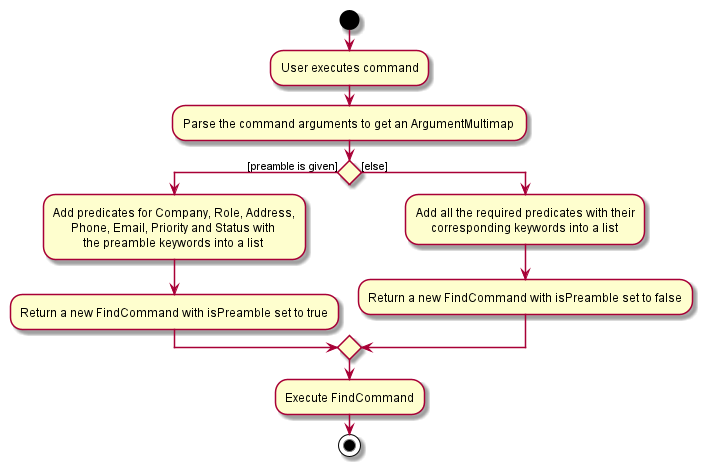
Design Considerations
Aspect: How to implement the different versions of Find command
-
Alternative 1 (current choice): Use a standardized command with the version to invoke determined by the type of user input parameters.
-
Pros: More streamlined, only one command.
This ensures that the user dont have to remember multiple command to use the different versions. -
Cons: Longer and less specific execute method.
-
-
Alternative 2: Use separate commands for the different versions of find.
-
Pros: More specific execute method for each of the command.
-
Cons: Makes the user remember more commands.
-
-
Alternative 3: Use the first word of the user input parameter to select which version of find command to invoke.
-
Pros: Slightly more streamlined than multiple commands.
This still requires user to remember the right words to invoke the different versions. -
Cons: Longer and less specific execution method.
-
Appendix A: Product Survey
Below are some of the programs currently available that could be used to manage internship applications, as well as their pros and cons
Huntr
Pros:
-
Uses online database
-
Uses kanban board for drag and drop management
Cons:
-
Cannot use CLI for interactions with the system
-
Cannot use without internet connection
-
Cannot use without signing up for an account
-
Cannot get filtered list, the whole board is always shown and can be disorganised
-
Cannot directly get reminders for deadlines, must add a new task
Excel
Pros:
-
Free for NUS students
-
Allows the user to define what to include
-
Allows the user to use it offline
Cons:
-
Does not use CLI for interactions with the system
-
Cannot easily go straight to managing internship applications, steep learning curve
-
Can get messy quickly, no inbuilt filter and archive functions
-
Does not include inbuilt statistics and reminder functions